springboot详细入门
2024-06-12 10:18
911
0
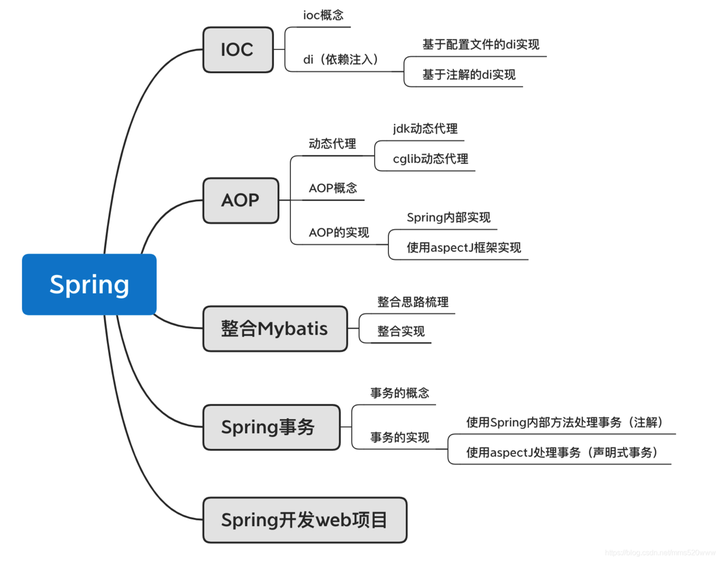
一、创建项目出现的问题
复制
问题1、application.yml文件无法识别
*解决方法:File->Settions->Plugins目录下 选中YAML,重启IDEA问题2、application.yml文件图标错误
*解决方法:File->Settings->File Types下 删除*.yml
- 1.
- 2.
- 3.
二、创建一个SpringBoot的项目
1 New Project
2 选择Spring Initializr
3 选择Web下的Web,然后Flish
4 导入依赖
复制
<!--SpringBoot起步依赖-->
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.5.RELEASE</version>
</parent>
<dependencies>
<!--web功能的起步依赖:SpringBoot集成SpringMVC进行Controller开发-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!--热部署-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
</dependency>
</dependencies>
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
IDEA设置,完成热部署
在Settings中找到Compiler,选中Build Project automatically
按Ctrl+Shift+Alt+/:选中compiler.automake.allow.when.app.running
底层分析:
复制
spring-boot-starter-parent:springboot起步依赖
在spring-boot-starter-parent中 resources资源引入: ${basedir}/src/main/resources下的 application*.yml application*.yaml application*.properties文件 在spring-boot-dependencies中 自动根据spring-boot-starter-parent的版本匹配相应的版本,进行了版本控制的作用 自动配置分析: @SpringBootApplication 标志该类是一个配置类:@Configration
- 1.
- 2.
三、SpringBoot整合Mybatis
第1步:导依赖:
- <!--mybatis起步依赖-->
- <dependency>
- <groupId>org.mybatis.spring.boot</groupId>
- <artifactId>mybatis-spring-boot-starter</artifactId>
- <version>2.0.1</version>
- </dependency>
- <!--MySQL依赖-->
- <dependency>
- <groupId>mysql</groupId>
- <artifactId>mysql-connector-java</artifactId>
- <version>5.1.6</version>
- </dependency>
第2步:yml配置文件
数据库配置spring:
配置Mybatis配置信息
spring集成Mybatis环境
pojo别名扫描包
复制
spring:
datasource:
driver-class-name: com.mysql.jdbc.Driver
url: jdbc:mysql://localhost:3306/springboot?useUnicode=true&characterEncoding=UTF-8&useSSL=false
username: root
password: 123456
mybatis:
type-aliases-package: com.zero.domain
mapper-locations: classpath:mapper/*Mapper.xml
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
第3步:创建实体
复制
package com.zero.domain;
public class User {
private Integer id;
private String name;
private String pass;
public Integer getId() {
return id;
} public void setId(Integer id) {
this.id = id;
} public String getName() {
return name;
} public void setName(String name) {
this.name = name;
} public String getPass() {
return pass;
} public void setPass(String pass) {
this.pass = pass;
} @Override
public String toString() {
return "User{" +
"id=" + id +
", name='" + name + '\'' +
", pass='" + pass + '\'' +
'}';
} }
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- 21.
- 22.
- 23.
- 24.
- 25.
第4步:创建接口
复制
package com.zero.mapper;
import com.zero.domain.User;
import org.apache.ibatis.annotations.Mapper;
import java.util.List;
@Mapper
public interface UserMapper {
public List<User> queryUserList();
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
第5步:创建映射文件
复制
<?xml version="1.0" encoding="utf-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.zero.mapper.UserMapper">
<select id="queryUserList" resultType="user">
SELECT * FROM demo </select>
</mapper>
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
第6步:测试
复制
package com.zero.controller;
import com.zero.domain.User;
import com.zero.mapper.UserMapper;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
//以json格式或字符串格式回写
@RestController
public class democontroller {
@Autowired
private UserMapper userMapper;
@RequestMapping("/quick")
public List<User> queryUserList(){
List<User> users = userMapper.queryUserList();
return users;
}
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
四、SpringBoot整合Spring Data JPA
第1步:导入依赖
复制
<!--spring-data-jpa-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<!--Mysql驱动-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.6</version>
</dependency>
<!--jdk9需要导入-->
<dependency>
<groupId>javax.xml.bind</groupId>
<artifactId>jaxb-api</artifactId>
<version>2.3.0</version>
</dependency>
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
第2步:创建实体,使用注解进行配置
复制
package com.zero.domain;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Integer id;
private String name;
private String pass;
public Integer getId() {
return id;
}public void setId(Integer id) {
this.id = id;
}public String getName() {
return name;
}public void setName(String name) {
this.name = name;
}public String getPass() {
return pass;
}public void setPass(String pass) {
this.pass = pass;
}@Override
public String toString() {
return "User{" +
"id=" + id +
", name='" + name + '\'' +
", pass='" + pass + '\'' +
'}';
}}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- 21.
- 22.
- 23.
- 24.
- 25.
- 26.
- 27.
- 28.
- 29.
- 30.
- 31.
- 32.
第3步:创建接口
复制
package com.zero.reposytory;
import com.zero.domain.User;
import org.springframework.data.jpa.repository.JpaRepository;
import java.util.List;
public interface UserReposytory extends JpaRepository<User,Integer> {
public List<User> findAll();
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
第4步:创建yml配置
复制
spring:
datasource:
driver-class-name: com.mysql.jdbc.Driver
url: jdbc:mysql://localhost:3306/springboot?useUnicode=true&characterEncoding=UTF-8&useSSL=false
username: root
password: 123456
#JPA Configuration
jpa:
database: MySQL
show-sql: true
generate-ddl: true
hibernate:
ddl-auto: update
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
第5步:测试
复制
package com.zero;
import com.zero.domain.User;
import com.zero.reposytory.UserReposytory;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.junit4.SpringRunner;
import java.util.List;
@RunWith(SpringRunner.class)
@SpringBootTest(classes = Demo614Application.class)
public class JpaTest {
@Autowired
private UserReposytory userReposytory;
@Test
public void test(){
List<User> all = userReposytory.findAll(); System.out.println(all);
}}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
五、Redis缓存
第1步:配置yml文件信息
复制
#redis
redis:
host: 127.0.0.1
port: 6379
- 1.
- 2.
- 3.
- 4.
第2步:测试
package com.zero;
复制
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.zero.domain.User;
import com.zero.reposytory.UserReposytory;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.test.context.junit4.SpringRunner;
import java.util.List;
@RunWith(SpringRunner.class)
@SpringBootTest(classes = Demo614Application.class)
public class RedisTest {
@Autowired
private RedisTemplate<String,String> redisTemplate;
@Autowired
private UserReposytory userReposytory;
@Test
public void test() throws JsonProcessingException {
//1.从Redis中获取数据,json字符串
String s = redisTemplate.boundValueOps("user.findall").get();
//2.判断Redis中是否存在想要的数据
if(null==s){
//3.1:不存在,从数据库中查询
List<User> all = userReposytory.findAll();
//3.2:将查询出的数据存储到Redis中
//通过web先将集合换换成json的字符串,使用Jackson进行转换
ObjectMapper ob = new ObjectMapper();
s = ob.writeValueAsString(all);
redisTemplate.boundValueOps("user.findall").set(s);
System.out.println("从数据库中获取数据");
}else {
System.out.println("从Redis缓存中获取数据");
}
//4.将数据打印在控制台
System.out.println(s);
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- 21.
- 22.
- 23.
- 24.
- 25.
- 26.
- 27.
- 28.
- 29.
- 30.
- 31.
- 32.
- 33.
- 34.
- 35.
- 36.
- 37.
- 38.
全部评论